One of the key tasks of a business application is the processing of financial transactions. In today's digital world, most transactions are made using payment systems, and the corporate accounting system must be able to work with this data. The 1C:Enterprise platform is intended for the development of corporate information systems and, accordingly, has broad integration capabilities with payment processing systems. Let's analyze the use of the REST API mechanism in 1C:Enterprise on the example of collaboration with the PayPal service.
In our example, we will make an application that receives the PayPal payment list. Further processing of payments in the information system (analysis of accounts receivable and payable, generation of reports, creation of documents necessary for the business process) can also be developed using the 1C platform, but this is another topic.
In the beginning, we need to register at https://developer.paypal.com.
After registration, PayPal creates two test accounts on which you can check how our application works:
By default, one personal account created, and the second is a business account. In the future, you can create additional accounts, if necessary. But for us, these two are enough for a start.
Now we can create the PayPal application (App):
Please also note that we make all the settings in the sandbox. Only after we debug our application, you can go to work in the main PayPal account.
After clicking the Create App button, a dialog opens, where we can enter the name of the PayPal application and indicate which test account should be associated with this application.
We select a test business account, and our application on the 1C platform will receive a list of payments from this account.
Then again click on Create App:
The settings page for the new application opens immediately.
First of all, the two fields are important here: Client ID and Secret. Using the values of these fields, we can connect from the program on the 1C platform using REST API and get the necessary data.
On the same page below, select the Transaction Search option. This option allows our program on the 1C platform to receive a list of operations. After that, click Save:
We can also log into our PayPal test accounts as in a regular account, see the balance, make a transfer of money from one test account to another, etc.
To enter the PayPal test account, you need to know its parameters. Here's how to do it:
Using the email and password, you can log into the test PayPal account.
Please note that you need to go to the address: https://www.sandbox.paypal.com/
Do not forget, we are working in test mode!
Well, in PayPal, we all set up, and now we are moving to the 1C platform. Let's get a list of operations.
Open the 1C configuration (you can open absolutely any) and create a new External data processor there:
Now we add six attributes: Host, GetTokenURL, ClientID, ClientSecret, access_token, app_id. Everyone has a value type - String (0):
We also add the tabular section PaymentList, and six additional attributes to it. In this tabular section, a list of payments will be displayed:
Create a new Form and open it:
We place the attributes on the form:
Add two commands to the form: GetToken and CallApiPayPal.
The first command will receive access token from PayPal, the second command will already receive a list of transactions.
&AtServer
Procedure OnCreateAtServer(Cancel, StandardProcessing)
Object.Host = "api.sandbox.paypal.com";
Object.GetTokenURL = "v1/oauth2/token";
Object.ClientID = "AQyoxGcnOkNjAnY7Hfuy3OZc4f8KUaYclYd8oVHs2CxXonjGKSQLUy675u42mVSPmwzmH5BWSHMSbhzy";
Object.ClientSecret = "EKCKWRA5_y3xSSlPpYqSWidqE6GlTE4TPvmgl0iSzaqvTlbPKRGu-cN1jdx6-wuvjGrfWO9blum6ec8W";
EndProcedure
The procedure defines the access parameters to the PayPal test account. This is filled in when creating the form, and it does not have to be specified every time.
Now create the program code for the command GetToken:
Function StringInBase64(DataString) Export
Stream = New MemoryStream;
Record = New DataWriter(Stream, TextEncoding.UTF8);
Record.WriteChars(DataString);
Record.Close();
BinaryData = Stream.CloseAndGetBinaryData();
StringFormatBase64 = Base64String(BinaryData);
StringFormatBase64 = StrReplace(StringFormatBase64, Chars.CR, "");// remove CR
StringFormatBase64 = StrReplace(StringFormatBase64, Chars.LF, "");// remove LF
Return StringFormatBase64;
EndFunction
&AtServer
Procedure GetTokenAtServer()
Host = Object.Host;
GetTokenURL = Object.GetTokenURL;
ClientID = Object.ClientID;
ClientSecret = Object.ClientSecret;
StrInput = ClientID + ":" + ClientSecret;
paramvalue = StringInBase64(StrInput);
HTTPRequest = New HTTPRequest;
HTTPRequest.ResourceAddress = GetTokenURL;
HTTPRequest.Headers.Insert("Authorization", Basic " + paramvalue);
HTTPRequest.Headers.Insert("Accept", "application/json");
HTTPRequest.Headers.Insert("Content-Type","application/x-www-form-urlencoded");
HTTPRequest.Headers.Insert("Accept-Language", "en_US");
HTTPRequest.Headers.Insert("Cache-Control", "no-cache");
HTTPRequest.SetBodyFromString("grant_type=client_credentials");
Connection = New HTTPConnection(Host,,,,,10,New OpenSSLSecureConnection);
JSONReader = New JSONReader;
Try
HTTPAnswer = Connection.Post(HTTPRequest); // post Request
JSONReader.SetString(HTTPAnswer.GetBodyAsString());
Data = ReadJSON(JSONReader, False);
Object.access_token = Data.access_token;
Object.app_id = StrReplace(Data.app_id, "APP-","");
If HTTPAnswer.StatusCode > 299 Then
ErrorText = "HTTPAnswer.StatusCode" + HTTPAnswer.StatusCode + "_PayPal API access token not received";
Raise ErrorText;
EndIf;
Except
ErrorText = "Sending PayPal_" + ErrorDescription();
EndTry;
EndProcedure // GetTokenAtServer()
&AtClient
Procedure GetToken(Command)
GetTokenAtServer();
EndProcedure
The code works very simply: HTTP request sent to the address "api.sandbox.paypal.com/v1/oauth2/token", ClientID and ClientSecret passed as parameters of this HTTP request.
In response, PayPal returns an access token to us and we save it:
Object.access_token = Data.access_token;
So, half we did - we got a token. Now let's create the code for the command “CallApiPayPal”.
&AtServer
Procedure CallPayPal()
If Not ValueIsFilled(Object.access_token) Then
GetTokenAtServer();
If Not ValueIsFilled(Object.access_token) Then
TextError = "Access token not received";
Raise TextError;
EndIf;
EndIf;
stringJSON = "";
Host = Object.Host;
dataBegin = Format(BegOfMonth(CurrentDate()), "DF=yyyy-MM-ddTHH:mm:ss") + "-0000";
dataEnd = Format(EndOfDay(CurrentDate()), "DF=yyyy-MM-ddTHH:mm:ss") + "-0000";
Url_Request = "/v1/reporting/transactions";
Url_Request = Url_Request + "?start_date=" + dataBegin + "&end_date=" + dataEnd + "&fields=all&page_size=500&page=1&sync_mode=false";
HTTPRequest = New HTTPRequest;
HTTPRequest.ResourceAddress = Url_Request;
HTTPRequest.Headers.Insert("Authorization", "Bearer " + Object.access_token);
HTTPRequest.Headers.Insert("Content-Type", "application/x-www-form-urlencoded");
HTTPRequest.SetBodyFromString(stringJSON);
Proxy = New InternetProxy(True);
Connection = New HTTPConnection(Host,,,,Proxy,10,New OpenSSLSecureConnection());
JSONReader = New JSONReader;
Try
HTTPResponse = Connection.Get(HTTPRequest); // Get Request
stringResponse = HTTPResponse.GetBodyAsString();
JSONReader.SetString(stringResponse);
dataResponse = ReadJSON(JSONReader, False);
If TypeOf(dataResponse) = Type("Structure")
AND dataResponse.Property("total_pages")
AND dataResponse.Property("page") Then
ProcessingPayPalData(dataResponse.transaction_details);
EndIf;
If HTTPResponse.StatusCode = 401 Then
// Request in PayPal API failed, 401 Not Authorized - get a new token
GetTokenAtServer();
CallPayPal();
ElsIf HTTPResponse.StatusCode > 299 Then
TextError = "HTTPResponse.StatusCode|" + HTTPResponse.StatusCode + "|Request in PayPal API failed";
Message = New UserMessage;
Message.Text = TextError;
Message.Message();
EndIf;
JSONReader.Закрыть();
Except
TextError = "Request in PayPal API failed|" + ErrorDescription();
Message = New UserMessage;
Message.Text = TextError;
Message.Message();
EndTry;
EndProcedure
&AtClient
Procedure CallApiPayPal(Command)
CallPayPal();
ThisForm.RefreshDataRepresentation();
EndProcedure
In this procedure, the code also works very simply, only the call occurs at the address "/v1/reporting/transactions". As parameters of the HTTP request, a token, start and end dates are used.
PayPal returns the JSON structure as an answer:
HTTPResponse = Connection.Get(HTTPRequest); // Get Request
stringResponse = HTTPResponse.GetBodyAsString();
JSONReader.SetString(stringResponse);
dataResponse = ReadJSON(JSONReader, False);
This structure has the parameter "transaction_details", which is an array that contains all the necessary information about transactions. This array processed in the procedure "ProcessingPayPalData":
ProcessingPayPalData(dataResponse.transaction_details);
Below is the code for this procedure:
&AtServer
Procedure ProcessingPayPalData(PayPalData)
Object.PaymentList.Clear();
For Each curElement In PayPalData Do
structureTransaction_Info = curElement.transaction_info;
structurePayer_Info = curElement.payer_info;
TransactionDate = structureTransaction_Info.transaction_updated_date;
structureTransactionAmount = Undefined;
structurePayerName = Undefined;
TransactionAmount = 0;
TransactionCurrency = "No currency";
TransactionSubject = "";
TransactionNote = "";
EmailAddress = "";
FullName = "";
If structureTransaction_Info.Property("transaction_amount",structureTransactionAmount) then
structureTransactionAmount.Property("currency_code", TransactionCurrency);
structureTransactionAmount.Property("value", TransactionAmount);
EndIf;
If Not structureTransaction_Info.Property("transaction_subject", TransactionSubject) Then
TransactionSubject = "";
EndIf;
If Not structureTransaction_Info.Property("transaction_note", TransactionNote) Then
TransactionNote = "";
EndIf;
TransactionSubject = TransactionSubject + " " + TransactionNote;
If Not structurePayer_Info.Property("email_address", EmailAddress) Then
EmailAddress = "No email";
EndIf;
If structurePayer_Info.Property("payer_name", structurePayerName) Then
If Not structurePayerName.Property("alternate_full_name", FullName) Then
FullName = "Nobody...";
EndIf;
Else
FullName = "Nobody...";
EndIf;
newPayment = Object.PaymentList.Add();
newPayment.date = TransactionDate;
newPayment.subject = TransactionSubject;
newPayment.amount = TransactionAmount;
newPayment.currency = TransactionCurrency;
newPayment.emailaddress = EmailAddress;
newPayment.fullname = FullName;
EndDo;
EndProcedure
The data array processed in a cycle, the necessary properties are filled (TransactionAmount, TransactionCurrency, TransactionSubject, etc.) and a new line added to the PaymentList - it will contain all the information about the transaction.
Well - let's check how our code works. Let's launch the 1C platform in an interactive mode, open our External Data Processor.
As you can see, all the necessary fields for access to REST API PayPal already filled. Excellent!
Now click the Get token button, and PayPal returns the access token to us:
Let's continue! Now we press the CallApiPayPal button and our list of transactions filled with data:
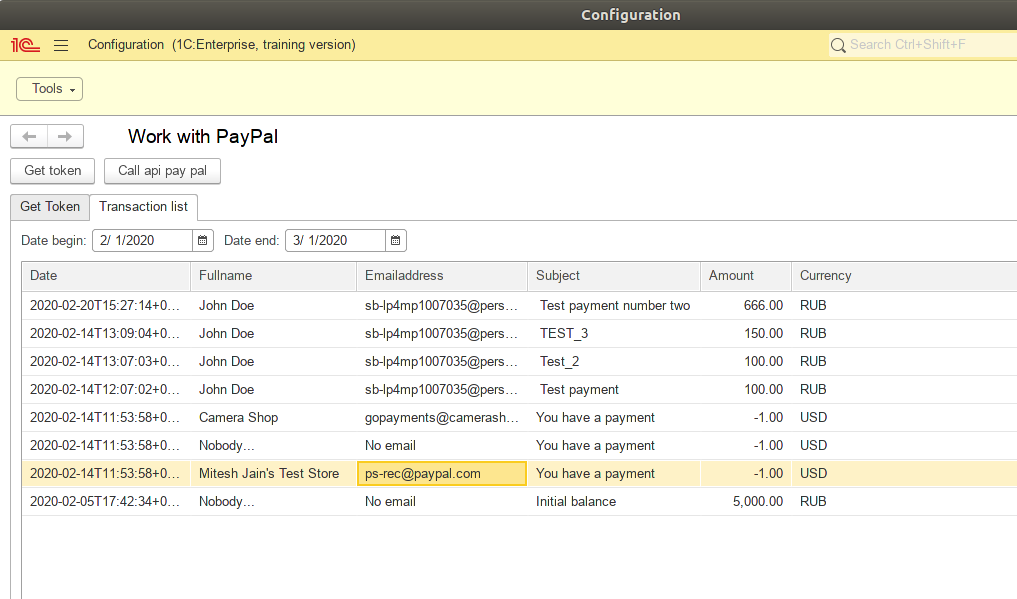
So, we got a list of transactions from PayPal using REST API!
You can download this Example for your own application.
Please, supplement your suggestions for the development of demo applications in our forum.
But this is not all the capabilities of the 1C platform. Stay with us - it will be more interesting further!